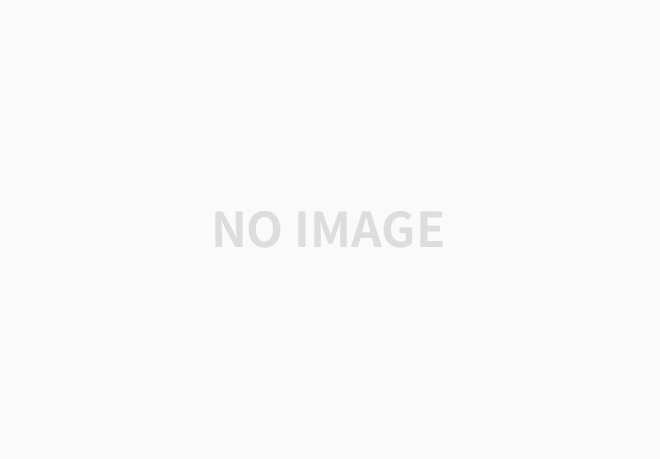
1. Embedding의 수학적 정의
두 vector space(벡터 공간) V와 E가 있을 때,
함수 f:V→E가 다음 조건을 만족하면 embedding이라고 정의됨.
- f는 단사 함수(injective function).
즉, 모든 v1,v2∈V에 대해:
f(v1)=f(v2)⇒v1=v2 - f 는 연속 함수(continuous function) 임.
- f의 Inverse function f−1:
- f(V)→V는 f(V)⊂E의 부분집합에서 V 로 가는 (부분적인) 함수임: f가 injective이지 surjective가 아니므로.
- Embedding의 정의에서 요구되는 건 E의 range에서만 정의되는 f−1 이 연속이어야 한다는 점임.
- 부분적으로 정의되는 역함수 면 충분함.
2021.09.14 - [.../Math] - Function (함수) : 간략 정의
Function (함수) : 간략 정의
Function은 흔히 mapping(사상), transformation(변환)이라는 용어로 불리기도 함. set으로 정의한다면, domain(정의역: 일종의 set)의 각 element에 대해 co-domain(공역: 역시 일종의 set)의 elements 중 오직 하나 로
dsaint31.tistory.com
2. 머신러닝에서의 응용
2-1. 단어 임베딩 (Word Embedding)
머신러닝, 특히 자연어 처리에서 Word Embedding(단어 임베딩)은 위의 수학적 정의를 다음과 같이 적용:
- V: 모든 가능한 words의 set (=사전, discrete set)
- E: n-차원 real vector space Rn (연속 공간)
- f:V→E, words를 embedding(=vector)로 mapping하는 function
사실, ML에서의 Embedding 은 저차원으로 매핑 과 함께 특정 관계(유사성)을 매핑한 공간에서도 유지시키면 되므로 1번의 엄격한 수학적 정의를 반드시 만족시키진 않음 (수학적 정의가 필요한 성질들을 어느정도 가이드해준다고 여겨도 됨).
Example: Word2Vec 모델
- king→f(vking)=[0.1−0.50.8…0.3]⊤∈R300
- queen→f(vqueen)=[0.2−0.40.7…0.4]⊤∈R300
이 때, function f는 다음 특성을 가짐:
- Injectivity: 서로 다른 단어는 서로 다른 벡터로 매핑함.
- continuous: 의미적으로 유사한 단어들은 벡터 공간에서 가까운 위치에 mapping함
2-2. 거리 보존 (Distance Preservation)
임베딩 f는
- 원래 공간 V의 "Semantic Distance(의미적 거리)"를
- 목표 공간 W의 유클리드 거리로 근사화 를 수행함:
dV(v1,v2)≈|f(v1)−f(v2)|2
where
- dV(v1,v2) 는 space V에서의 v1,v2 간의 semantic distance function.
이를 통해 Embedding f는
"왕"과 "여왕" 사이의 관계가 "남자"와 "여자" 사이의 관계와 유사함을
다음과 같이 벡터 연산으로 표현할 수 있음:
f(v킹)−f(v남)+f(v여)≈f(v퀸)
Example of distance function:
2023.07.23 - [.../Math] - [ML] Cosine Similarity
[ML] Cosine Similarity
ML에서 주로 다루는 데이터는 바로 vector이다. (matrix도 vector들이 결합하여 이루어진 것이라고 생각할 수 있음.) Cosine Similarity는 두 vector가 얼마나 유사한지(similar)를 측정하기 위한 metric 중 하나로
dsaint31.tistory.com
3. 딥러닝에서의 구현
신경망에서 임베딩 층(Embedding Layer)은 다음과 같이 구현됩니다:
E:V→RdE(v)=W⊤v
where,
- W∈R(|V|×d)는 Trainable Weight Matrix 임.
- |V| 는 사전에 포함된 words의 수를 의미
- the set of words에 해당하는 V의 cardinality (set에 포함된 elements의 수)
- one-hot encoding을 사용하는 input vector의 component수.
- d : embedding 의 차원 (= output dimensionality)
이 Embedding Layer 는
- backpropagation을 통해 학습
- 특정 task의 loss function을 최소화하는 방향으로 학습이 이루어짐.
3-1. PyTorch
PyTorch의 nn.Embedding
레이어는 기본적으로 선형 변환 만을 수행.
- 구현:
- 학습 후 주어진 입력 index에 대응하는 embedding vector (dense vector)를 반환.
- 입력 index에 대응하는 Embedding Matrix의 row를 조회(lookup)하여 반환.
- 활성화 함수: 포함되어 있지 않음
import torch.nn as nn embedding_layer = nn.Embedding( num_embeddings, # the total num of unique items (input) embedding_dim, # ndim of embeddings )
3-2. Keras (TensorFlow)
Keras의 Embedding
레이어 역시 기본적으로 선형 변환 만을 수행.
- 구현:
- 학습 후 주어진 입력 index에 대응하는 embedding vector (dense vector)를 반환.
- PyTorch와 유사하게 Embedding Matrix에서 해당 인덱스의 row vector를 조회하여 반환.
- 활성화 함수: 포함되어 있지 않음
from tensorflow.keras.layers import Embedding embedding_layer = Embedding( input_dim, # size of dictionary + 1 (0번 index포함) output_dim, # ndim of embeddings )
3-3. 비선형성 추가 방법
두 프레임워크 모두 Embedding 레이어 자체에는 Non-Linear Activation 함수가 포함되어 있지 않음
비선형성을 추가하려면 다음과 같은 방법을 사용할 수 있음:
- Embedding 레이어 뒤에 별도의 Activation Function Layer 추가
- 커스텀 Embedding 레이어 구현
- 모델 구조에서 Embedding 레이어 다음에 Dense 와 Activation Function 추가
위의 방법에서 Activation Function은
non-linear여야 비선형성이 추가됨.
같이보면 좋은 자료들
https://gist.github.com/dsaint31x/21354c4eb74686ad432b1eb1bf64dc59
ML_Embedding.ipynb
ML_Embedding.ipynb. GitHub Gist: instantly share code, notes, and snippets.
gist.github.com
2024.09.28 - [Programming/ML] - [ML] Embedding
[ML] Embedding
0. Embedding의 수학적 정의2024.09.28 - [Programming/ML] - [ML] Embedding의 수학적 정의 및 Embedding Layer [ML] Embedding의 수학적 정의 및 Embedding Layer1. Embedding의 수학적 정의두 vector space(벡터 공간) V와 E가 있
dsaint31.tistory.com
'Programming > ML' 카테고리의 다른 글
[ML] scikit-learn: Custom Transformer (2) | 2024.10.01 |
---|---|
[ML] Embedding (0) | 2024.09.28 |
[ML] Kernel Function 이란: Kernel Trick 포함 (0) | 2024.09.28 |
[ML] Radial Basis Function Kernel (RBF Kernel) (1) | 2024.09.26 |
[ML] Ensemble 기법 (0) | 2024.09.08 |