bar chart 그리기 : error bar 포함
Axes object의 ax
의 bar
method를 이용하여 bar chart를 그릴 수 있음.
기본 사용법
bar
method의 기본적인 사용법은 다음과 같음.
ax.bar( x_pos, # 각 bar가 그려질 x축 위치를 item으로 가지는 ndarray. data, # x_pos 의 item 에 대한 bar의 높이에 해당하는 데이터들. width = 0.8, # bar width. bottom = None, align='center', alpha=0.5, # bar 스타일 관련. yerr=error, # error bar를 기재할 때 사용됨. ecolor='black', capsize=10, # errorbar의 color와 capsize 지정. )
x_pos
:- bar chart의 x 좌표들을 item으로 가지는 ndarray. 반드시 필요.
data
:- bar의 높이에 해당하면,
xpos
와 같은 수의 item을 가져야 함.(순서대로 대응됨)
- bar의 높이에 해당하면,
width
:- bar의 넓이 기본은
0.8
임.
- bar의 넓이 기본은
bottom
:- bar의 아래쪽 위치.
None
이 기본으로0
부터 막대의 높이까지 그려지게 됨.bottom
으로 어디서부터 막대가 그려질지 지정가능.
align
:x_pos
의 각 itme의 위치에 bar가 그려지는데 align을 어떻게 할지를 지정함.center
(기본값),edge
,leading
,tailing
중에서 고를 수 있음.
color
: bar의 색.alpha
: 투명도edgecolor
: bar의 테두리 색.linewidth
: 선 두께yerr
:- error bar의 크기를 가짐.
data
와 마찬가지로xpos
와 같은 item의 수를 가져야 함.
예제코드
다음은 bar chart를 그리는 간단한 예제 코드임. (error bar포함)
import numpy as np import matplotlib.pyplot as plt # Enter raw data a = np.array([6.4e-5 , 3.01e-5 , 2.36e-5, 3.0e-5, 7.0e-5, 4.5e-5, 3.8e-5, 4.2e-5, 2.62e-5, 3.6e-5]) b = np.array([4.5e-5 , 1.97e-5 , 1.6e-5, 1.97e-5, 4.0e-5, 2.4e-5, 1.9e-5, 2.41e-5 , 1.85e-5, 3.3e-5 ]) c = np.array([3.3e-5 , 1.2e-5 , 0.9e-5, 1.2e-5, 1.3e-5, 1.6e-5, 1.4e-5, 1.58e-5, 1.32e-5 , 2.1e-5]) # Calculate the average a_mean = np.mean(a) b_mean = np.mean(b) c_mean = np.mean(c) # Calculate the standard deviation a_std = np.std(a) b_std = np.std(b) c_std = np.std(c) # Create lists for the plot labels = ['A', 'B', 'C'] x_pos = np.arange(len(labels)) data = [a_mean, b_mean, c_mean] error = [a_std, b_std, c_std] # Build the plot fig, ax = plt.subplots() ax.bar( x_pos, data, yerr=error, align='center', alpha=0.5, ecolor='black', capsize=10, ) ax.set_ylabel('m$^{-1}$') ax.set_xticks(x_pos) ax.set_xticklabels(labels) ax.set_title('Bar chart with error bars') ax.yaxis.grid(True) # Save the figure and show plt.tight_layout() # plt.savefig('bar_plot_with_error_bars.png') plt.show()
결과는 다음과 같음
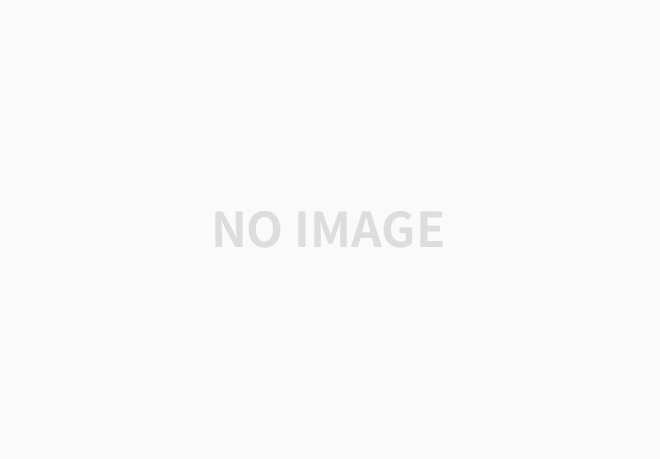
더 읽어보면 좋은 자료들
해당 코드의 gist URL: https://gist.github.com/dsaint31x/4f48d8fa2d3fa5d1f4efde764d4e7b59
matplot_barchart.ipynb
matplot_barchart.ipynb. GitHub Gist: instantly share code, notes, and snippets.
gist.github.com
errobar관련 좋은 튜토리얼 : https://pythonforundergradengineers.com/python-matplotlib-error-bars.html
Bar charts with error bars using Python and matplotlib
Bar charts with error bars are useful in engineering to show the confidence or precision in a set of measurements or calculated values. Bar charts without error bars give the illusion that a measured or calculated value is known to high precision or high c
pythonforundergradengineers.com
'Programming' 카테고리의 다른 글
[colab] google drive와 colab연동하기 (기초) (0) | 2023.09.14 |
---|---|
[ML] Ward’s linkage method (0) | 2023.08.06 |
[Python] for statement (0) | 2023.07.30 |
[PyQt6] QSizePolicy 설정. (0) | 2023.07.03 |
[Python] Regular Expression : re 요약 (0) | 2023.07.03 |